Basics
Contents
History
C++ was created in the early 80's by Bjarne Stroustrup at Bell Labs. It is a general purpose object oriented programming language used in a variety of different application. Let us study how a computer program is executed on a computer. The diagram is somewhat simplistic and offers an overview of how a computer will work in general.See full image
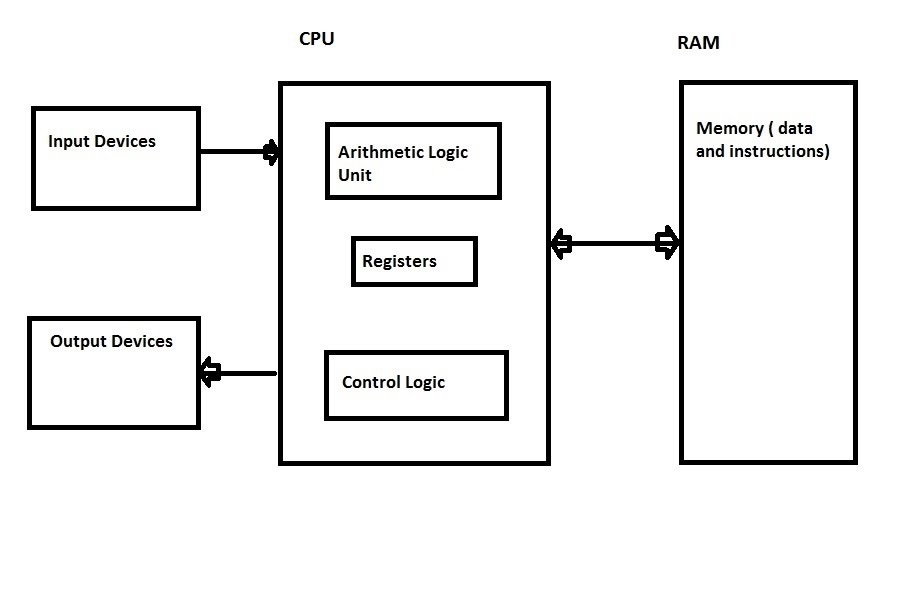
The instructions for the program are stored in the RAM and the CPU grabs an instruction from the RAM and executes it. It will then grab the next instruction and execute that and so on. What type of instructions are these ? Well these are the instructions that the CPU understands. In case of an Intel CPU the machine language is based on x86 . That is the only thing the CPU understands. It does not know about C++ , Java or any other language. It only knows about the x86 machine language. What do the instructions of this machine language look like
000000 00001 00010 00110 00000 100000 100011 00011 01000 00000 00001 000100As you can imagine writing machine language instructions is a tedious and impractical task. Programmers used Assembly language instead to do their programming. So how did the CPU run Assembly language . Well it didn't. The Assembly language program was converted to machine language by a compiler. What did the Assembly language instructions look like ?
MOV AL, 1h ; Load AL with immediate value 1 MOV CL, 2h ; Load CL with immediate value 2 MOV DL, 3h ; Load DL with immediate value 3The Assembly language instruction had one to one correlation with machine language and was slightly better in terms of using opcodes but still a tedious task. So now the high level languages came such as Fortran, Pascal, C . For a long time programming was done in these high level languages . These language had features like functions, procedures , structures. A programmer could use variables and did not have to worry about mapping variables to specific addresses in memory. However these languages involved the programmer converting the application problem in terms of the language which still bore a close relationship to the way the CPU worked. The concept of object oriented languages was developed and thus came languages such as Java, C# and C++ . Now a programmer could translate the application problem in terms of objects and classes. The way we think about problems and concepts can be correlated and organized better with object oriented programming. As an example think of an application for the bank . We can think of a customer with properties such as name, age, gender, address, phone. This customer could have an account and the account could be checking or saving. An account can have properties like amount and account number. It could also have methods like add a deposit and so on. This sort of technique can be used in many problems where we can model the solution in terms of classes to more closely resemble the problem domain. C++ is based on the "C" language that was created by Dennis Ritchie in the late 60's. The "C" language was a procedural based language . Object oriented features were added to it and C++ was born. C++ is a super set of the "C" language and most "C" programs can run under "C++". Both the languages involve a steep learning curve.
Compiling and setting up environment
We are going to write our first program, compile and run it under different operating systems. As is the convention our first program is going to print "Hello World" to the console and that's all it does.Windows
On the Windows operating system we have many options in order to compile and run it.Cygwin
One option is to install the Cygwin software from the site:https://cygwin.com
Cygwin is a Unix simulator that runs as an application on Windows. Once you start Cygwin it opens a dos like command prompt window and you feel as if you are on ta Unix system. Choose the "g++" compiler and the "make" utility from the options when installing. For a text editor we have many options available . One editor is "Textpad" that can be downloaded from the site:https://textpad.com
You should also download the syntax definition file from the "Textpad" site for C++ language. That will enable the syntax coloring .File: hello.cpp
#include <iostream> using namespace std ; int main() { cout << "Hello World." << endl ; }
Save this to a file. You can name it to be "hello.cpp" but it can be anything you like. Then from the "Cygwin" command line, navigate to the folder where you created "hello.cpp" and type in: g++ hello.cpp Then do a "ls" and you should see a file "a.exe" or "a.out" . This is the executable that got created. We can run this executable by the command : ./a.out and you should see the following output on the console: Hello World
Eclipse and Netbeans
Other IDE environments that can be installed are Eclipse and Netbeans but these require a compiler such as "g++" to be installed separately. Microsoft has an IDE called "Visual Studio Express" that can be downloaded for free. There are some restrictions on this particular version but it can be used for learning purposes.Microsoft Visual Studio
Microsoft has Visual Studio that is a tool that can be used to compile different languages including C++ . They are supposed to have a free edition of the the Visual Studio. If you have access to a remote Unix system then we can connect to it and use it to compile our programs. . Winscp is a utility that can be used to access files on the remote server. https://winscp.net/eng/download.php Snapshot showing the Winscp Session/New Session settings. Choose the "Save" to save your settings including the password.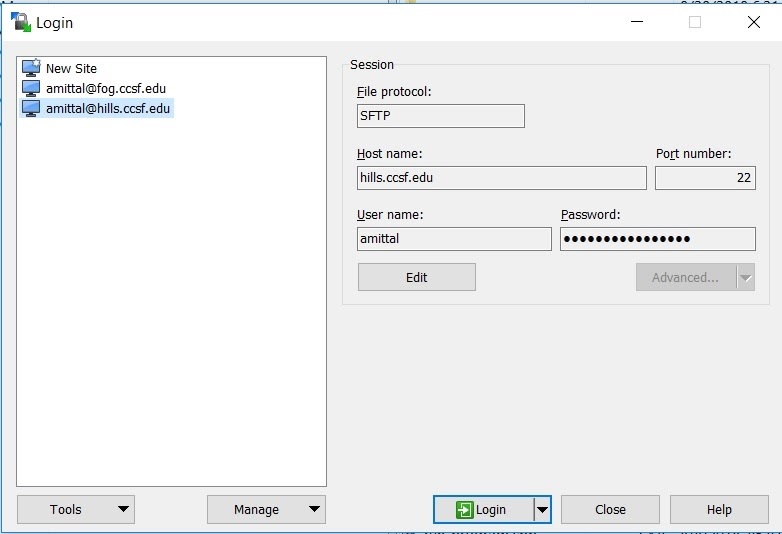
We can also integrate an editor such as Textpad using the "Options/Preferences" dialog box:
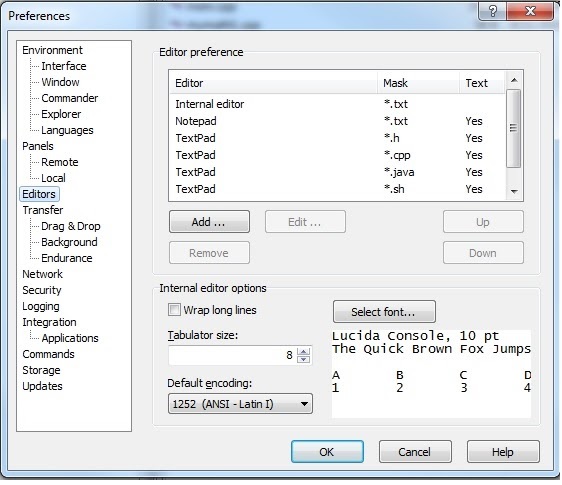
Putty
https://the.earth.li/~sgtatham/putty/latest/w64/putty.exeThe program "putty.exe" is a single file that be saved to a folder such as "C:\putty" . We can integrate Putty with WinScp by changing the "Option/Preferences" screen .
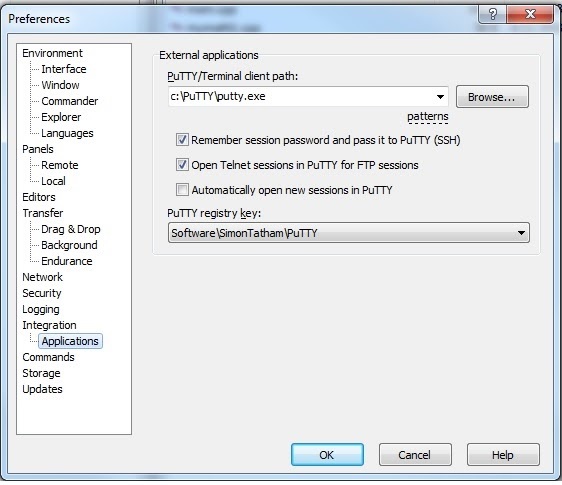
The checkbox "Remember session password will allow you to open up Putty from WinScp without a prompt for password.
Apple
On the Mac there is software by Apple called Xcode . You need an Apple developer account and it's free. There are 2 versions of it. One includes an IDE and the other is command line based. You can also access a remote unix system. In the below example we show how to access a remote system called then you can use the Hills from the "Terminal" window.[amittal@fog ~]$ ssh amittal@hills.ccsf.edu
Enter your password and now you are in the Hills system. To create your files you can use the "vi", "vim" or "nano" editor. You can read about these editors on the web. You can also use "sftp" to transfer files from your Mac to the Hills server if you want through the Terminal window.
Unix
Unix systems vary in the setup of the C++ compiler. Usually one can check for the existence of the "g++" compiler by typing:g++
on the command line. If you get an error of the sort "input file not found" the C++ compiler is installed and if you get "command not found" error then the g++ compiler is not installed.
Compilation
We have seen that if we have a C++ program:File: hello.cpp
#include <iostream> using namespace std ; int main() { cout << "Hello World." << endl ; }
g++ hello.cpp
In the above command we have not specified an executable so the C++ compiler produces the default executable with a name of "a.out" that we can run with the command.
./a.out
We need to place the "./" in front of the executable name to tell Unix to look for the executable in the current folder instead of in the environment path. We can specify the name of the executable using the "-o" option.
g++ -o hello hello.cpp
./hello
We can also compile to an executable using object files. Let's study that in the next section.
Object and Executable files
We can compile to an object format using the "-c" option.g++ -c hello.cpp
This will produce a file named "hello.o" . This is an object file containing machine language instructions. However it is not an executable. We cannot run it.
[amittal@hills object_1]$ ./hello.o
-bash: ./hello.o: Permission denied
Even if we change the permissions on the file
chmod 777 hello.o
and try to run it again we get:
[amittal@hills object_1]$ ./hello.o
-bash: ./hello.o: cannot execute binary file
The compiler does not check if the file has a main method or if it has function definitions. The compiler makes sure that the syntax is correct and then compiles to machine language instructions.
[amittal@hills object_1]$ g++ -c hello1.cpp
[amittal@hills object_1]$ g++ hello1.o
/lib/../lib64/crt1.o: In function `_start':
(.text+0x20): undefined reference to `main'
collect2: error: ld returned 1 exit status
After compiling if we try to link the object file and create an executable file then the C++ compiler complains about not finding the "main" method.
File: hello2.cpp
#include <iostream> using namespace std ; int add( int num1, int num2 ) ; int main() { add( 3 , 4 ) ; cout << "Hello World." << endl ; return(0) ; }
[amittal@hills object_1]$ g++ -c hello2.cpp
[amittal@hills object_1]$ g++ hello2.o
hello2.o: In function `main':
hello2.cpp:(.text+0xf): undefined reference to `add(int, int)'
collect2: error: ld returned 1 exit status
[amittal@hills object_1]$
We have declared a function "add" but not provided a definition for it. We are able to compile and produce an object file for it because the rule for a file compilation in C++ is that the file only needs a declaration in order for the file to get compiled . However when we try to create the executable then the compiler will check if the definition exists somewhere and if it doesn't then it will complain. Both object files and executable files contain machine language code but an object file does not need a "main" method and it does not need a definition of a function.
Multiple Files
A C++ project will often consist of more than 1 file. How do we compile multiple files in C++ ? Assume we have 2 files: "hello2a.cpp" and "add2a.cpp" .File: hello2a.cpp
#include <iostream> using namespace std ; //Function add int add( int num1, int num2 ) { return num1 + num2 ; } int main() { int ret = add( 3 , 4 ) ; cout << ret << endl ; return(0) ; }
File: add2a.cpp
//Function add int add( int num1, int num2 ) { return num1 + num2 ; } Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ g++ hello2a.cpp add2a.cpp Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ ./a.exe 7 We can also create the executable using object files. Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ g++ -c add2a.cpp Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ g++ -c hello2a.cpp Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ g++ hello2a.o add2a.o Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ ./a.exe 7
Make Utility
Once you are on the Unix system a utility called "make" can also be used to compile your program. We can create entries in a text file called "Makefile" . As an example to compile the "hello1.cpp" program we can use:File: Makefile
hello.exe: hello1.cpp g++ -o hello.exe hello1.cppThe first item on the line "hello.exe" is the target. That is the file we want to end up with. To the right of that we have the dependency which is "hello1.cpp" and below that we have the command to build the target. The second line should have tabs before it. The "make" utility is very particular about the tabs and will not accept spaces. So what's the advantage of the "makefile" ? Well for one you do not have to type the whole command in. You can just type "make"
Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ make g++ -o hello.exe hello1.cpp Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro $ make make: 'hello.exe' is up to date. Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/introand that will get the compilation process going. Let's run the "make" command again. Notice it does not compile the "hello1.cpp" again. The tool "make" will first check for the existence of "hello.exe". If it did not exist then it knows it has to run the command. In this case it exists so it looks at the dependency and sees that it is a file but not a target. If it were a target then it would evaluate the target. If it found that the target needed evaluation then the command would be run. In this the "hello.cpp" is not a target but a simple file. The tool checks if this file has been changed since the last time of compilation. It hasn't so we get a message that "hello.exe" is up to date. Now we make a superficial change to "hello1.cpp" and run make again. Now the make tool will see that the dependency has changed since the last time of compilation and run the "g++" command again.
We can also write the Makefile so that it works with object files instead. What's an object file ? We can direct the C++ compiler to compile to an object file instead of an executable with the following command:
g++ -c hello.cpp
This produces a file called "hello.o" . This is also a machine language file but is not an executable. We can't run it. The "-c" option does not force the compiler to look for a "main" method. To produce an executable we run the "g++" command again but use an object file instead of a ".cpp" file.
g++ hello.o
This will then produce an executable that we can actually run. Why do we need this object file approach to begin with. Can't we just compile the ".cpp" files every time ? Compiling a single file may not make much of a difference but consider a large project consisting of many files. Let's say our project consists of 5 files and we compile an executable using the command:
g++ first1.cpp first2.cpp first3.cpp first4.cpp first5.cpp
Now if we change the "first1.cpp" then using the above command all the files must be compiled again. That means the compiler has to check the grammar and syntax errors and then compile each file to machine code and then link them together to produce the executable file. Using object files approach we can do:
g++ -c first1.cpp
g++ -c first2.cpp
...
g++ -c first5.cpp
This will produce the files:
first1.o first2.o first3.o first4.o first5.o
Then we can link them together to produce the executable file.
g++ first1.o first2.o first3.o first4.o first5.o
Let's see what happens if we change the code in first1.cpp . We do not need to recompile the rest of the files. We can compile the first1.cpp with the command:
g++ -c first1.cpp
and then link the files together:
g++ first1.o first2.o first3.o first4.o first5.o
The process of linking is very fast as the files have already been compiled to machine language code.
Let's see how we would do the above process using the make utility for 2 files:
File: hello2.cpp
File: add2.cpp
File: m1.txt
hello2.exe: hello2.o add2.o g++ -o hello2.exe hello2.o add2.o hello2.o: hello2.cpp g++ -c hello2.cpp add2.o: add2.cpp g++ -c add2.cppThe dependency can be a target as in the above case. The dependencies will be evaluated and if the "make" utility determines that there has been a change in the dependency then it will run the command to create "hello2.exe" . We place the above commands in a file called "m1.txt". The make file does not have to be named "Makefile". We can use the "-f" option to specify a makefile by a different name.
Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro/make $ make -f m1.txt g++ -c hello2.cpp g++ -c add2.cpp g++ -o hello2.exe hello2.o add2.oThe make tool first checks if "hello2.exe" exists. It does not so it know it has to run the command "g++ -o hello2.exe hello2.o add2.o" . But first the dependencies need to be evaluated. The "hello2.o" is a target for the second task. The tool evaluates this task. The target "helllo2.o" does not exist. The dependency is a file so the command "g++ -c hello2.cpp" is executed. In a similar manner the task "add2.o" is evaluated. Once the dependecies are done then the main command is run. What happens if we change "add2.cpp" ? The same process repeats as before. The "hello2.o" exists and it's dependency is not changed. So we skip this step and go to "add2.o". This task is evaluated and so the main command is run again. Anytime the dependency changes the main command is run. The "make" utility does not know anything about "C++" but it knows about timestamps,targets, commands and we can use these to compile even complicated, large projects.
C++Versions
https://github.com/AnthonyCalandra/modern-cpp-featuresC++98 C++03 C++11 Move semantics, lambdas, auto, thread C++14 Variable templates C++17 Std variant, optional C++20 Template syntax lambdasThe version numbers correspond to the year that the version was released. The newer versions are from C++ 11 onwards. The "C++ 11" is the most important and we shall try to cover all it's features.
Structure of a C++ program
File: hello.cpp
#include <iostream> using namespace std ; int main() { cout << "Hello World." << endl ; }
Every C++ program must have a main method. That is where the execution starts from. Any functions or variables that are used from the library must be declared. That is where the "include" files come in.
Declarations
If we use a function in a cpp file then it must be defined or declared before we can use it.File: d1.cpp
#include <iostream> using namespace std ; int sum( int x1, int x2 ) { return x1+x2 ; } int main() { cout << sum( 2, 3 ) << endl ; }
Output: 5 The above compiles fine. However let's now place the function sum below the main function.
File: d2.cpp
#include <iostream> using namespace std ; int main() { cout << sum( 2, 3 ) << endl ; } int sum( int x1, int x2 ) { return x1+x2 ; }
Output:
$ g++ d2.cpp d2.cpp: In function ‘int main()’: d2.cpp:9:11: error: ‘sum’ was not declared in this scope 9 | cout << sum( 2, 3 ) << endl ;
Compilation starts from the top for a cpp file. Also the compilation for a single cpp file is independent of the compilation of other files in the project if there are other files. From the top it finds the function "sum" getting used but hasn't encountered the declaration or the definition and thus the compiler throws an error. If we have a functions such as:
int sum( int x1, int x2 ){ return x1+x2 ;}
Then the declaration is the signature of the function:
int sum( int x1, int x2 )
and the definition is the above functions with the code. All we need for the compiler is to tell it what the declaration looks like:
File: d3.cpp
#include <iostream> using namespace std ; int sum( int x1, int x2 ) ; int main() { cout << sum( 2, 3 ) << endl ; } int sum( int x1, int x2 ) { return x1+x2 ; }
Portability
Let's say we compile the above program on Windows and we get an exe named "hello.exe" . Can we take this "exe" and run it say on a Mac or Windows machine ? No we can't. Even if the processors are the same ( Intel compatible ) an operating system has it's own format for executable s . Specific operating system information is inserted into the executable making it impossible to run on other systems. This is evident when we have to download programs for specific Unix systems. Instead of giving the executable to download the website will usually provide the source files along with the make file.Namespace
Namespaces are used to prevent name conflicts between variables, functions. A namespace can be thought of as enclosing a set of variables or function names into a block that has a label. This is especially important when global variables come into play. Let's say we have the following program "namespace1.cpp" :File: namespace1.cpp
#include <iostream> using namespace std ; //Global variable int var1 = 100 ; int main() { //local variable hides the global variable int var1 = 5 ; cout << var1 << endl ; }
File: namespace2.cpp
#includeUsing namespace for functions. We create 3 files: main.cpp , mymath1.cpp , mymath1.husing namespace std ; //Global variable namespace group1 { int var1 = 100 ; } int main() { //local variable hides the global variable int var1 = 5 ; cout << group1::var1 << endl ; } Output: 100
File: main.cpp
#include "mymath1.h" #includeusing namespace std ; using namespace mine ; int main() { int result = doSum( 4, 5 ) ; cout << result << endl ; return 1 ; }
File: mymath1.cpp
#include "mymath1.h" namespace mine { int doSum( int x1, int x2 ) { return ( x1 + x2 ) ; } }
File: mymath1.h
namespace mine { int doSum( int x1, int x2 ) ; }If we have a namespace for a function in the ".cpp" file then we need to place the namespace in the heade file also.
namespace mine { int doSum( int x1, int x2 ) ; }
Using namespace for integers in header files.
We create 3 files:
main2.cpp , mymath2.cpp , mymath2.h
File: main2.cpp
#include "mymath2.h" #includeusing namespace std ; using namespace mine ; int main() { cout << g1 << endl ; return 1 ; }
File: mymath2.cpp
namespace mine { int g1 = 100; }
File: mymath2.h
namespace mine { extern int g1 ; ; }
Note that it's possible to define the same namespace in multiple cpp files.
main3.cpp , mymath3.cpp , mymath3.h
File: main3.cpp
#include "mymath2.h" #includeusing namespace std ; using namespace mine ; namespace mine { extern int g1 ; int g2 = 20 ; } int main() { cout << g1 << endl ; cout << g2 << endl ; return 1 ; }
File: mymath3.cpp
namespace mine { int g1 = 100; }
File: mymath3.h
namespace mine { extern int g1 ; ; } $ g++ main3.cpp mymath3.cpp ; ./a.exe 100 20
C++ uses the namespace "std" to enclose the objects and functions in it's standard library.
File: namespace3.cpp
#includeWe get an error because the cout is in a namespace. We can correct it either by placing the//using namespace std ; int main() { cout << "Testing." ; } $ g++ namespace3.cpp namespace3.cpp: In function ‘int main()’: namespace3.cpp:6:9: error: ‘cout’ was not declared in this scope; did you mean ‘std::cout’? 6 | cout << "Testing." ;
using namespace std ;at the top or using "std::cout" . The phrase "using namespace" states that everything in that namespace is accessible without putting the namespace in front of the variable or the function name. Though this is convenient if we are using the variable in the namespace multiple times it can create a problem if we have a variable of the same name.
File: namespace4.cpp
#includeIn the above if we don't put a "std::" in front of the cout then there is a name conflict.using namespace std ; int main() { // Introduces a nane conflict int cout ; std::cout << "Testing." ; }
Gdb
The Unix g++ compiler also has a debugger facility. Let's take the below program and use the "gdb" debugger with it.File: main.cpp
#includeusing namespace std ; int main() { int i1 = 5 ; i1 = i1 + 2 ; i1 = i1 + 3 ; i1 = i1 + 4 ; return 1 ; }
To work with the debugger we need to use different compiler option. g++ -g main.cpp and to run the gdb: gdb ./a.out We can then set break points: break 11 The command to step through is: step The command to run is: run We can also print values with the print command: print i1 The debugger in an IDE will be much easier to use in terms of the graphical interface than the gdb. However gdb provides the functionality that one expects from a normal GUI debugger.
Extern
We have 2 files:File: main.cpp
int main() { g1 = 5 ; }
File: mymath1.cpp
//Global Variable int g1 ;
We know that if we try to compile the 2 files with the command: main.cpp:7:2: error: ‘g1’ was not declared in this scope We receive an error of the form: main.cpp:7:2: error: ‘g1’ was not declared in this scope We can't use the same technique that we used for the functions because if we do:
File: main1.cpp
int g1 ; int main() { g1 = 5 ; }Then we are defining the variable "g1" again . We need some way to declare but not define the "g1" variable. This is where the "extern" type comes in. Now our "main2.cpp" becomes:
File: main2.cpp
extern int g1 ; int main() { g1 = 5 ; }
Preprocessor
The C++ compiler compiles a ".cpp" file to machine code. This process consists of 2 parts. The first is where the preprocessor part of compiler modifies your ".cpp" file and the second part is where it converts the modified file to machine code. All the preprocessor statements start with the hash "#" character. Lets take an example of how this works. We can have our program spread over multiple files. Let's take an example.File: main.cpp
#includeusing namespace std ; int main() { int result = doSum( 4, 5 ) ; cout << "Hello World" << endl ; return 1 ; }
File: mymath1.cpp
int doSum( int x1, int x2 ) { return ( x1 + x2 ) ; }
Now we compile the 2 files to create an executable: g++ -o main.exe main.cpp mymath1.cpp However running the above command produces the error: main.cpp: In function ‘int main()’: main.cpp:11:28: error: ‘doSum’ was not declared in this scopeThis error is happening because we need to tell "main.cpp" the signature of the function. What are the parameters that "doSum" takes and what value does it return ? This is called a "declaration" . Adding this declaration produces the following code:
File: main1.cpp
#includeusing namespace std ; int doSum( int x1, int x2 ) ; int main() { int result = doSum( 4, 5 ) ; cout << "Hello World" << endl ; return 1 ; }
Now the compilation succeeds: g++ -o main.exe main1.cpp mymath1.cppThe file "main.cpp" is compiled first and the compiler makes sure that the function "doSum" is used properly. It does not have the full definition of the function yet because that is in another file "mymath1.cpp" . The compiler will create an object file internally for "main.cpp" . Right now it has not verified that the "doSum" has actually a definition but it will do that later when linking. Now it compiles "mymath1.cpp" to an object file. Now there are 2 object files and the linking process begins. The process of linking takes the 2 object files and will check if the declared functions have the definitions for them and also whether the function "main" exists. It will then create an executable.
What if some other file in our project wanted to use the
"doSum" function? We would have to include the declaration
for the function. We can do this in a better way by using
header files. We can place the declaration in the header
file and name the header file "mymath.h" .
File: main2.cpp
#include#include "mymath.h" using namespace std ; int main() { int result = doSum( 4, 5 ) ; cout << "Hello World" << endl ; return 1 ; }
File: mymath1.cpp
int doSum( int x1, int x2 ) { return ( x1 + x2 ) ; }
File: mymath.h
int doSum( int x1, int x2 ) ;
Deller@DESKTOP-DNEP4MC /cygdrive/c/WebSite/Learn/2/cplusplus/intro/preprocessor $ g++ -o main.exe main2.cpp mymath1.cppThe header file is processed by the preprocessor and a new "main.cpp" file is created that contains the contents of the header file.
Binary Arithmetic
We work with numbers using base 10 or the decimal system. When we have a number such as 245 This is interpreted as : 2 * 10 to power 2 + 4 * 10 to power 1 + 5 If we have a base such as 10 then the digits range from 0 to 9 ( 0 to base -1 ) . When we go from right to left we multiply the digit by the base to the power increasing the power by 1 each time. Computer work with base 2 also called the binary system. The digits are 0 and 1 . The number 101 is 5 in decimal system( 1*2 power2 + 0 + 1 ) . If we have 3 digits then the unsigned number will look like below: 000 -- 0 001 -- 1 010 -- 2 011 -- 3 100 -- 4 101 -- 5 110 -- 6 111 -- 7 Two's complement. A negative binary number is represented in two's complement. In this scheme the leftmost bit is "1" and represents the negative number. The right most bits are inverted and then a "1" is added to get the negative number amount. Ex: 101 The bit "01" are inverted to form "10" and then a "1" is added to form "11" so the number "101" is actually "-3" . If we have 3 digits then the signed numbers look as below: 000 0 001 1 010 2 011 3 100 -4 101 -3 110 -2 111 -1 Base 16 also called Hexadecimal system . The digits are 0-9, A-F with A representing 10 and F representing 15. Examples: F0 - 240 AF - 175
File: bin1.cpp
#includeusing namespace std ; int main() { unsigned char b1 = 254 ; b1 = b1 + 1 ; cout << (int)b1 << endl ; b1 = b1 + 1 ; cout << (int)b1 << endl ; } Output: $ ./a.exe 255 0 An unsigned char is 8 bits whose range is 0 to 255 . The variable "b1" is initialized to 254 and that gets incremented to 255 and that is printed out. Now we add 1 to 255. 1111 1111 0000 0001 ------------- 1 0000 0000 The carry over of 1 is discarded because we only have 8 bits to work with. and we get a 0 . Now let's change the unsigned char to a char.
File: bin2.cpp
#include254 is: 1111 1110 Adding 1 to it is 1111 1111 and this is -1 . Adding 1 to this produces a 0 .using namespace std ; int main() { char b1 = 254 ; b1 = b1 + 1 ; cout << (int)b1 << endl ; b1 = b1 + 1 ; cout << (int)b1 << endl ; } Output: $ ./a.exe -1 0
Variables
A valid variable must have as the first character the letter a to z or A to Z or the underscore character "_" . After the first character; a character can be a to z or A to Z or a digit from 0 - 9 or the underscore. In C++ uppercase and lowercase characters represent unique names and can't be interchanged. The name "score" is different from the name "Score" .Data Types
Below are the primitive data types: Integer Character Boolean Floating Point Double Void Wide Char A data type can have additional modifiers. As an example the "Integer" data type can have the following modifiers: Signed Unsigned Short Long A program that prints the size of different types.
File: size1.cpp
#includeusing namespace std; int main() { cout << "Size of char : " << sizeof(char) << " byte" << endl; cout << "Size of int : " << sizeof(int) << " bytes" << endl; cout << "Size of short int : " << sizeof(short int) << " bytes" << endl; cout << "Size of long int : " << sizeof(long int) << " bytes" << endl; cout << "Size of signed long int : " << sizeof(signed long int) << " bytes" << endl; cout << "Size of unsigned long int : " << sizeof(unsigned long int) << " bytes" << endl; cout << "Size of float : " << sizeof(float) << " bytes" < Output: Size of char : 1 byte Size of int : 4 bytes Size of short int : 2 bytes Size of long int : 8 bytes Size of signed long int : 8 bytes Size of unsigned long int : 8 bytes Size of float : 4 bytes Size of double : 8 bytes Size of wchar_t : 4 bytesExercises
1)
File: intro_ex1.cpp
#include <iostream> using namespace std ; namespace outer1 { namespace inner1 { int var1 = 100 ; } } int main() { cout << var1 << endl ; return 0 ; }Correct the error in the above code. Use the prefix method and the using method. The nested namespace can be referenced using the notation "outer1::inner1" .
2)
File: intro_ex2.cpp
#include <iostream> #include <limits.h> using namespace std; int main() { char ch1 ; cout << "Min value of char is:" << CHAR_MIN << endl ; cout << "Max value of char is:" << CHAR_MAX << endl ; return 0 ; }Run the above program and explain the output. What are the limits of an unsigned char type ? Check your answer by adding 2 more lines and using the constans UCHAR_MIN and UCHAR_MAX .
Editing
If we are on a Windows system and we installed the WinScp utlility then we can edit the files on the hills server directly. However it is important to be able to edit files using the console. There are 2 modes. One is the command mode and the other edit mode. We use the command mode to save, delete copy paste and the edit mode to type in characters.Create a folder called "edit" and then the command. $ vi 1.txtWe use the "i" key to enter the edit mode. Type in a few lines and then hit the "Esc" key. After that hit the ":" key and type in "wq" ( w to write and q to quit ) . A useful resource is the vi cheat sheet at:
vi cheat sheetgeneral commands
Now that we can create files with some contents here are some more commands. More information can be found using the "man command" . The "man" ( manual ) displays information about the command.
pwd The "pwd" ( Present Working Directory ) tells us where we are.[amittal@hills ~]$ pwd /users/amittalcd The "cd" can be used to change directories. Assume we are in the home folder on hills and there is a folder inside it called "test".[amittal@hills ~]$ cd test [amittal@hills ~]$ pwd /users/amittal/test To go back up we can use the command "cd ..": [amittal@hills ~]$ cd .. [amittal@hills ~]$ pwd /users/amittal and to go to the home folder from any folder we can use cd by itself. [amittal@hills ~]$ cd [amittal@hills ~]$ pwd /users/amittal we can also use the full path name to go to a folder. [amittal@hills ~]$ cd /users/amittal/testls The "ls" can be used to list files.$ ls a.exe data.txt data1.txt data2.txt hw2.cpp hw2s.cpp The "ls -l" shows the detailed listing. $ ls -l total 87 -rwxrwxr-x+ 1 Deller None 76434 Jun 5 09:47 a.exe -rw-rw-r--+ 1 Deller None 128 Jun 5 08:53 data.txt -rw-rw-r--+ 1 Deller None 128 Jun 5 09:47 data1.txt There are many options with the "ls" command. As an example "ls -ltr" displays the long list sorted by date from oldest to the newest. $ ls -ltr total 87 -rw-rw-r--+ 1 Deller None 128 Jun 5 08:53 data.txt -rwxrwx---+ 1 Deller None 1674 Jun 5 09:09 hw2s.cpp -rwxrwxr-x+ 1 Deller None 76434 Jun 5 09:47 a.exe -rw-rw-r--+ 1 Deller None 128 Jun 5 09:47 data1.txt -rw-rw-r--+ 1 Deller None 93 Jun 5 09:47 data2.txt -rwxrwx---+ 1 Deller None 842 Jun 5 10:09 hw2.cpp -rw-rw-r--+ 1 Deller None 0 Jun 6 02:25 1.txtmkdir The "mkdir" can be used to create folders.$ mkdir test1 If the folder is empty we can remove it with the "rmdir" command: $ rmdir test1 Otherwise we can use "rm -r" . $ mkdir test1 $ cd test1 $ touch 1.txt $ cd test1 $ cd .. $ rmdir test1 rmdir: failed to remove 'test1': Directory not empty $ rm -r test1 We can also use the "rm" command to remove files.cp The "cp" can be used to copy files.$ cp char2.cpp char3.cpp $ ls a.exe char1.cpp char2.cpp char3.cpp strings.htmlcat The "cat" command will list the contents of a file. We can use it to list the contents of multiple files also. $ cat 1.txt 2.txt date Prints the current date and time. [amittal@hills edit]$ date Sat Dec 23 19:46:21 PST 2023 clear The "clear" command clears the console and brings the cursor to the top of the console. echo The "echo" command can print a string on the console. [amittal@hills edit]$ echo "This is a unix class." This is a unix class. It can also print values of variables ( we shall study the variables in more details later on ) . [amittal@hills edit]$ echo $PATH /usr/local/bin:/usr/bin:/usr/local/sbin: /usr/sbin:/sbin:/users/amittal/.local/bin: /users/amittal/bin tar The "tar" command can be used to compress and extract files. To compress the folder "mymath" to a file called "mymath.gz" tar -czvf mymath.gz mymath To extract the compressed file use: The "c" option means create a tar file. The "z" option means filter the archive through gzip. Use "gzip" to compress. The "v" option means verbose. The "f" option means specufy the archive file. tar -xvf mymath.gz This will create a folder "mymath" in the current directory and extract all the files there. The "x" option means extract. who The "who" command shows the list of users who are logged in the system. The related command "whoami" shows the current user who is logged in. [amittal@hills ~]$ whoami amittal wc [amittal@hills 1]$ cat 1.txt This is a unix class. [amittal@hills 1]$ wc 1.txt 1 5 22 1.txt The "wc" lists the lines, words and characters in the file. find [amittal@hills cs160a]$ find . -name "1.txt" ./hw1/first/1.txt ./hw1/first/second/1.txt ./hw1/second/1.txt ./edit/1.txt ./1/1.txt The find command can be used to search for files. In the example we are stating that the search start from the current folder ( "." ) and that the name of file should be "1.txt" . grep The "grep" command searches for a string. If we give the command and the string then "grep" expects the input from the console. If there is a match then the line is printed out again. $ grep cat The cat is sleeping. The cat is sleeping. The dog eats food. In the below the command "grep" looks in the file "3.txt" and prints the lines that contain the word "cat" . $ cat 3.txt The cat is sleeping. The dog eats food. $ grep "cat" 3.txt The cat is sleeping.