Stack and Heap
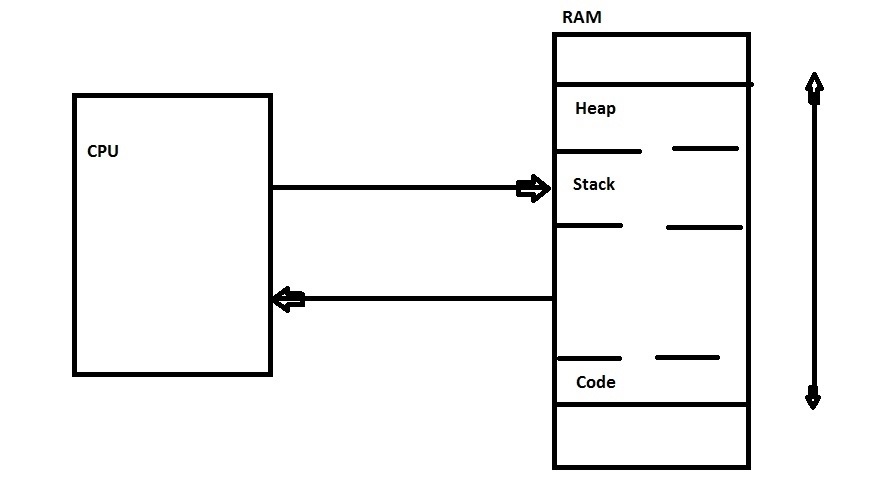
Video on stack and heap
A program can be run by executing a file such as:
./a.out
The operating system will allocate some area in the RAM for the program to be run. The code of the exe ( machine language instructions ) will occupy some space and 2 sections in the RAM will be reserved ; the stack and the heap. When a program is run the CPU will fetch an instruction from the RAM and process it and then fetch another instruction from the RAM and process it . Complications arise when there is a function to be called. Assume the following are machine language instructions loaded in the RAM .
int sum( int x1 , int x2 ) //A return ( x1 + x2 ) //B int main() int result ; //C result = sum( 4 , 6 ) ; //DAssume the current instruction is at "C". Now we need to execute the function "sum" but the "sum" is another section of the code. Somehow we need to tell that function that once we are done we need to come back to the main function. So we store this location on the stack.
Stack --------- //D Store this locationNow we also need to pass the values 4 and 6 to the function . So let's store those also in the stack.
Stack --------- 6 4 //D Store this locationNow we tell the CPU that the next instruction is A and we store 4 and 6 on the stack. Now the CPU starts executing the code at the location "sum" and the sum function grabs the numbers 4 and 6 and has the result 10 that it needs to give back to where the function was called in the "main" function. How does it do that ? Again it puts it on the stack:
Stack --------- 10It uses the //D location and control is transferred to the main function where the code picks up the result 10 from the stack and and assigns it to the variable "result" . The area for storing this information for a particular function is called a stack frame. Local variables are also created and stored on the stack.
Ex: void function1() { int x1 ; int x2 ; int x3 ; } Stack --------- x3 x2 x1The requirement is that the size of the variables must be known at compile time of the variables to be allocated on the stack. The "heap" is used to store memory that has been created dynamically when the program is run. The size of the memory can be dynamic. There are 2 functions that can be used to do this:
"malloc" and "new"
Memory allocated in any other way will be allocated on the stack. When the program exits or is terminated by the user then the whole block of memory is released by the operating system.
File: stack1.cpp
File: stack2.cpp
File: heap1.cpp
File: heap2.cpp